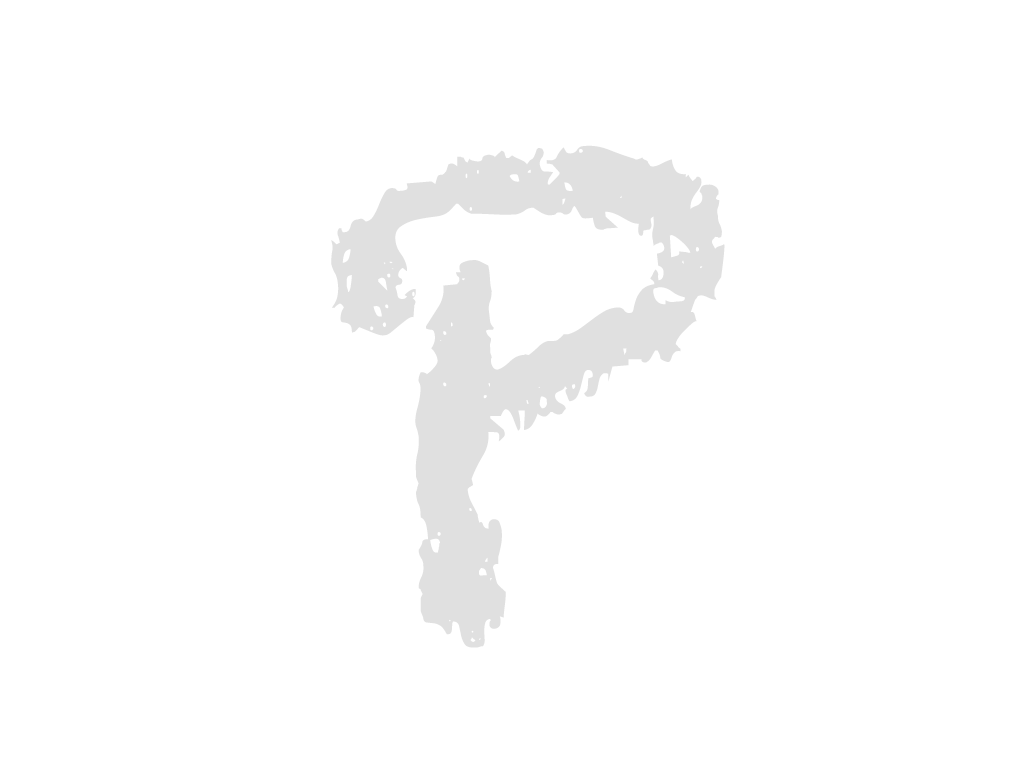
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
import React, {useRef, useCallback, useMemo, useContext} from 'react';
import {View, StyleSheet, Text} from 'react-native';
import {Polyline, Marker} from 'react-native-maps';
import {BottomSheetModal, BottomSheetModalProvider} from '@gorhom/bottom-sheet';
import {MapContext} from '../context/MapContext';
import Map from '../component/Map';
import DriveStatus from './../component/DriveStatus';
import Button from '../component/Button';
import CurrentMarker from '../resoureces/files/images/current_location.png';
import {pageTitle, marginBottom} from '../resoureces/styles/GlobalStyles';
import Camera from '../component/Photo';
import {PRIMERY, WHITE} from '../color';
export default function Driving({navigation}) {
const {nodes, location, km, setSearchQuery, setNodes} =
useContext(MapContext);
//node 마지막 좌표 구하기
const nodesEndPoint = nodes.slice(-1);
const resultEndPoint = nodesEndPoint[0];
//바텀시트
const bottomSheetModalRef = useRef(null);
const snapPoints = useMemo(() => ['25%'], []);
// callbacks
const handleSheetChanges = useCallback(index => {
console.log('handleSheetChanges', index);
}, []);
const handlePresentModalPress = useCallback(() => {
bottomSheetModalRef.current?.present();
}, []);
const handleClosePress = useCallback(() => {
setSearchQuery(null);
setNodes([]);
bottomSheetModalRef.current?.close();
navigation.navigate('Main');
}, []);
return (
<BottomSheetModalProvider>
<View style={styles.container}>
<Map
lat={location.latitude}
long={location.longitude}
latDelta={0.001}
longDelta={0.001}
followsUserLocation={true}>
<Marker
coordinate={location}
width={50}
height={50}
image={CurrentMarker}
/>
<Marker coordinate={resultEndPoint} />
<Polyline
coordinates={nodes}
strokeWidth={10}
strokeColor={PRIMERY}
/>
</Map>
<Camera />
<DriveStatus distance={km} onPress={handlePresentModalPress} />
<BottomSheetModal
ref={bottomSheetModalRef}
index={0}
snapPoints={snapPoints}
onChange={handleSheetChanges}>
<View style={styles.contentContainer}>
<Text style={[pageTitle, marginBottom]}>
운행을 종료 하시겠습니까?
</Text>
<Button
title={'운행종료'}
padding={10}
backgroundColor={PRIMERY}
color={WHITE}
width={'100%'}
textAlign={'center'}
onPress={handleClosePress}
/>
</View>
</BottomSheetModal>
</View>
</BottomSheetModalProvider>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
backgroundColor: 'grey',
},
contentContainer: {
flex: 1,
alignItems: 'center',
padding: 16,
},
image: {
width: '100%',
height: '100%',
flex: 1,
},
});