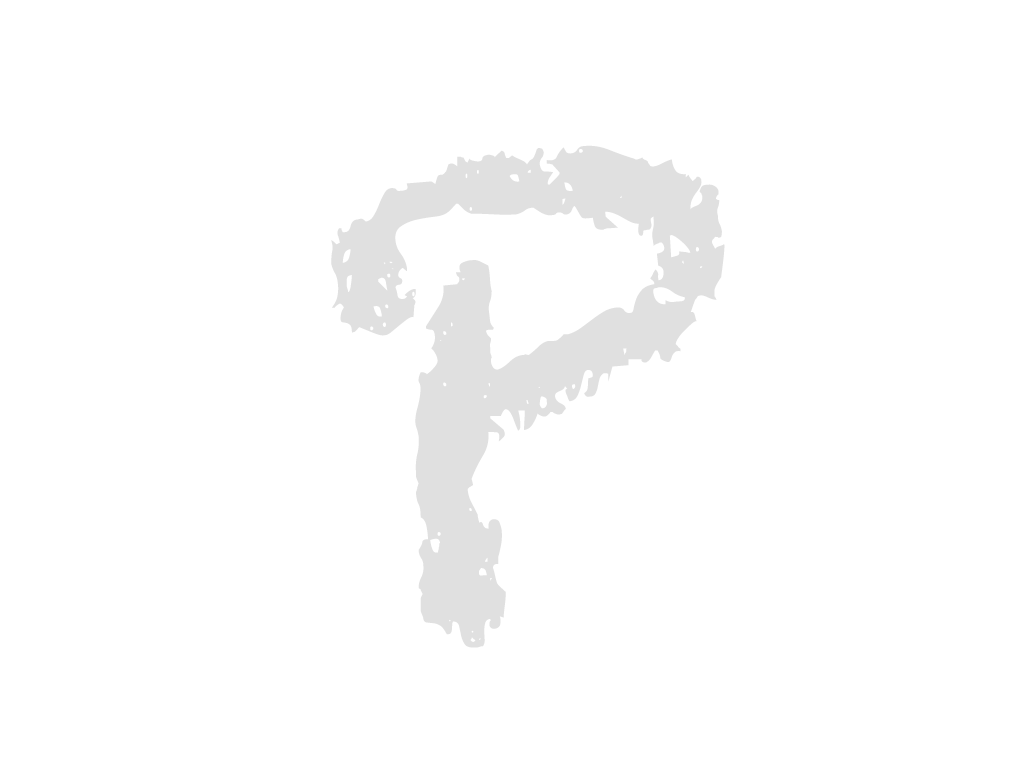
--- src/main/java/com/takensoft/common/file/service/Impl/FileServiceImpl.java
+++ src/main/java/com/takensoft/common/file/service/Impl/FileServiceImpl.java
... | ... | @@ -4,9 +4,14 @@ |
4 | 4 |
import com.takensoft.common.file.service.FileService; |
5 | 5 |
import com.takensoft.common.file.vo.FileVO; |
6 | 6 |
import com.takensoft.common.util.JWTUtil; |
7 |
+import com.takensoft.common.util.ResponseData; |
|
7 | 8 |
import lombok.RequiredArgsConstructor; |
8 | 9 |
import org.egovframe.rte.fdl.cmmn.EgovAbstractServiceImpl; |
9 | 10 |
import org.springframework.beans.factory.annotation.Value; |
11 |
+import org.springframework.http.HttpHeaders; |
|
12 |
+import org.springframework.http.HttpStatus; |
|
13 |
+import org.springframework.http.MediaType; |
|
14 |
+import org.springframework.http.ResponseEntity; |
|
10 | 15 |
import org.springframework.stereotype.Service; |
11 | 16 |
import org.springframework.transaction.annotation.Transactional; |
12 | 17 |
import org.springframework.util.StringUtils; |
... | ... | @@ -15,6 +20,7 @@ |
15 | 20 |
|
16 | 21 |
import java.io.File; |
17 | 22 |
import java.io.IOException; |
23 |
+import java.nio.charset.Charset; |
|
18 | 24 |
import java.nio.file.Paths; |
19 | 25 |
import java.util.*; |
20 | 26 |
import java.util.UUID; |
... | ... | @@ -55,6 +61,11 @@ |
55 | 61 |
for (MultipartFile file : multipartFileList) { |
56 | 62 |
if (file.isEmpty()) { |
57 | 63 |
continue; |
64 |
+ } |
|
65 |
+ |
|
66 |
+ int size = Long.valueOf(file.getSize()).intValue() / 100000; |
|
67 |
+ if (size > 200) { |
|
68 |
+ return -1; |
|
58 | 69 |
} |
59 | 70 |
|
60 | 71 |
// 절대 경로 생성 |
... | ... | @@ -98,14 +109,14 @@ |
98 | 109 |
file.transferTo(uploadFile); |
99 | 110 |
} catch (IOException e) { |
100 | 111 |
e.printStackTrace(); |
101 |
- return result; |
|
112 |
+ return 0; |
|
102 | 113 |
} |
103 | 114 |
|
104 | 115 |
// DB등록 |
105 | 116 |
result += fileDAO.fileInsert(fileVO); |
106 | 117 |
} catch (IOException e) { |
107 | 118 |
e.printStackTrace(); |
108 |
- return result; |
|
119 |
+ return 0; |
|
109 | 120 |
} |
110 | 121 |
} |
111 | 122 |
|
--- src/main/java/com/takensoft/portal/entDscsnAply/web/EntDscsnAplyController.java
+++ src/main/java/com/takensoft/portal/entDscsnAply/web/EntDscsnAplyController.java
... | ... | @@ -51,6 +51,10 @@ |
51 | 51 |
responseData.setStatus(HttpStatus.OK); |
52 | 52 |
responseData.setMessage("정상적으로 문의 등록되었습니다."); |
53 | 53 |
return new ResponseEntity<>(responseData, headers, HttpStatus.OK); |
54 |
+ } else if(insertResult < 0) { |
|
55 |
+ responseData.setStatus(HttpStatus.PAYLOAD_TOO_LARGE); |
|
56 |
+ responseData.setMessage("업로드 가능한 파일 용량 제한을 초과했습니다."); |
|
57 |
+ return new ResponseEntity<>(responseData, headers, HttpStatus.PAYLOAD_TOO_LARGE); |
|
54 | 58 |
} else { |
55 | 59 |
if (result.containsKey("writeCount") && result.containsKey("lastDatetime")) { |
56 | 60 |
responseData.setStatus(HttpStatus.TOO_MANY_REQUESTS); |
... | ... | @@ -131,6 +135,10 @@ |
131 | 135 |
responseData.setStatus(HttpStatus.OK); |
132 | 136 |
responseData.setMessage("정상적으로 처리되었습니다."); |
133 | 137 |
return new ResponseEntity<>(responseData, headers, HttpStatus.OK); |
138 |
+ } else if(result < 0) { |
|
139 |
+ responseData.setStatus(HttpStatus.PAYLOAD_TOO_LARGE); |
|
140 |
+ responseData.setMessage("업로드 가능한 파일 용량 제한을 초과했습니다."); |
|
141 |
+ return new ResponseEntity<>(responseData, headers, HttpStatus.PAYLOAD_TOO_LARGE); |
|
134 | 142 |
} else { |
135 | 143 |
responseData.setStatus(HttpStatus.BAD_REQUEST); |
136 | 144 |
responseData.setMessage("요청 적용에 실패하였습니다.\n담당자에게 문의하세요."); |
--- src/main/java/com/takensoft/portal/entInfo/web/EntInfoController.java
+++ src/main/java/com/takensoft/portal/entInfo/web/EntInfoController.java
... | ... | @@ -66,6 +66,10 @@ |
66 | 66 |
responseData.setMessage("정상적으로 등록 처리되었습니다."); |
67 | 67 |
responseData.setData(result); |
68 | 68 |
return new ResponseEntity<>(responseData, headers, HttpStatus.OK); |
69 |
+ } else if(insertResult < 0) { |
|
70 |
+ responseData.setStatus(HttpStatus.PAYLOAD_TOO_LARGE); |
|
71 |
+ responseData.setMessage("업로드 가능한 파일 용량 제한을 초과했습니다."); |
|
72 |
+ return new ResponseEntity<>(responseData, headers, HttpStatus.PAYLOAD_TOO_LARGE); |
|
69 | 73 |
} else { |
70 | 74 |
responseData.setStatus(HttpStatus.INTERNAL_SERVER_ERROR); |
71 | 75 |
responseData.setMessage("등록에 실패하였습니다.\n담당자에게 문의하세요."); |
... | ... | @@ -151,6 +155,10 @@ |
151 | 155 |
responseData.setStatus(HttpStatus.OK); |
152 | 156 |
responseData.setMessage("정상적으로 기업정보 수정 처리되었습니다."); |
153 | 157 |
return new ResponseEntity<>(responseData, headers, HttpStatus.OK); |
158 |
+ } else if(result < 0) { |
|
159 |
+ responseData.setStatus(HttpStatus.PAYLOAD_TOO_LARGE); |
|
160 |
+ responseData.setMessage("업로드 가능한 파일 용량 제한을 초과했습니다."); |
|
161 |
+ return new ResponseEntity<>(responseData, headers, HttpStatus.PAYLOAD_TOO_LARGE); |
|
154 | 162 |
} else { |
155 | 163 |
responseData.setStatus(HttpStatus.BAD_REQUEST); |
156 | 164 |
responseData.setMessage("기업정보 등록 실패하였습니다.\n담당자에게 문의하세요."); |
--- src/main/java/com/takensoft/portal/ivstDscsn/web/IvstDscsnController.java
+++ src/main/java/com/takensoft/portal/ivstDscsn/web/IvstDscsnController.java
... | ... | @@ -52,6 +52,10 @@ |
52 | 52 |
responseData.setMessage("정상적으로 투자상담 등록되었습니다."); |
53 | 53 |
responseData.setData(result); |
54 | 54 |
return new ResponseEntity<>(responseData, headers, HttpStatus.OK); |
55 |
+ } else if(insertResult < 0) { |
|
56 |
+ responseData.setStatus(HttpStatus.PAYLOAD_TOO_LARGE); |
|
57 |
+ responseData.setMessage("업로드 가능한 파일 용량 제한을 초과했습니다."); |
|
58 |
+ return new ResponseEntity<>(responseData, headers, HttpStatus.PAYLOAD_TOO_LARGE); |
|
55 | 59 |
} else { |
56 | 60 |
responseData.setStatus(HttpStatus.INTERNAL_SERVER_ERROR); |
57 | 61 |
responseData.setMessage("투자상담 등록에 실패하였습니다.\n담당자에게 문의하세요."); |
... | ... | @@ -126,6 +130,10 @@ |
126 | 130 |
responseData.setStatus(HttpStatus.OK); |
127 | 131 |
responseData.setMessage("정상적으로 투자상담 수정 처리되었습니다."); |
128 | 132 |
return new ResponseEntity<>(responseData, headers, HttpStatus.OK); |
133 |
+ } else if(result < 0) { |
|
134 |
+ responseData.setStatus(HttpStatus.PAYLOAD_TOO_LARGE); |
|
135 |
+ responseData.setMessage("업로드 가능한 파일 용량 제한을 초과했습니다."); |
|
136 |
+ return new ResponseEntity<>(responseData, headers, HttpStatus.PAYLOAD_TOO_LARGE); |
|
129 | 137 |
} else { |
130 | 138 |
responseData.setStatus(HttpStatus.BAD_REQUEST); |
131 | 139 |
responseData.setMessage("투자상담 수정에 실패하였습니다.\n담당자에게 문의하세요."); |
--- src/main/java/com/takensoft/portal/rvwMttr/web/RvwMttrController.java
+++ src/main/java/com/takensoft/portal/rvwMttr/web/RvwMttrController.java
... | ... | @@ -54,6 +54,10 @@ |
54 | 54 |
responseData.setMessage("정상적으로 검토사항 등록 처리되었습니다."); |
55 | 55 |
responseData.setData(result); |
56 | 56 |
return new ResponseEntity<>(responseData, headers, HttpStatus.OK); |
57 |
+ } else if(insertResult < 0) { |
|
58 |
+ responseData.setStatus(HttpStatus.PAYLOAD_TOO_LARGE); |
|
59 |
+ responseData.setMessage("업로드 가능한 파일 용량 제한을 초과했습니다."); |
|
60 |
+ return new ResponseEntity<>(responseData, headers, HttpStatus.PAYLOAD_TOO_LARGE); |
|
57 | 61 |
} else { |
58 | 62 |
responseData.setStatus(HttpStatus.INTERNAL_SERVER_ERROR); |
59 | 63 |
responseData.setMessage("검토사항 등록에 실패하였습니다.\n담당자에게 문의하세요."); |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?